Create JSON Data API in SAP to integrate with C# application Part – 1
Create JSON Api in SAP ERP to integrate SAP with C# Application
This tutorial will help you to create JSON Api in SAP ERP to integrate SAP with C# Application. This Technics will help you to connect SAP with out using any third party tools. You can READ, INSERT, UPDATE and DELETE data as per your requirements.
Step by step to create API in SAP Object Class
Step 1 : Create a Y or Z table in SAP to store data using T-Code SE11. Here we are using the Table name ZAPI_TESTUSER. Data Elements AD_SMTPADR, AD_NAMEFIR and AD_NAMELAS are SAP Standard Data Elements. You can create Data Elements as per your requirements.
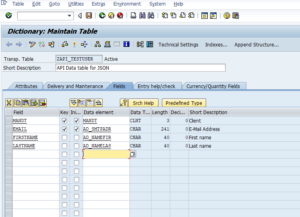
Step 2 : Configure Technical Settings and Enhancement Category for the table and activated the Table. Use $TMP package and Local Object. If you want to transport the request to your PRD server then you can make transport request.

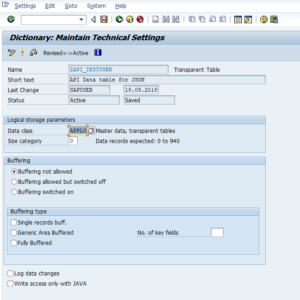

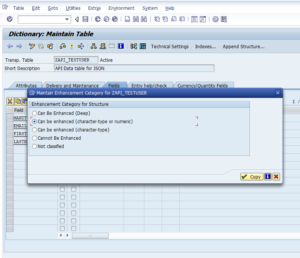
Step 3 : Create a Table Type using T-Code SE11 and select Table Type when asked for Type selection. I use the name ZTT_TESTUSER and selected the type Table Type. In Line Type field add the table name which we created earlier ie.ZAPI_TESTUSER. Now we created Table and Table Type in SAP.

Step 4 : Create an Object Class using T-Code SE24 and here we use the name ZAPI_TEST as Object Name. Add IF_HTTP_EXTENSION in Interfaces and then press enter.Add a Public Attribute STATUS with Associated Type STRING.
Step 5 : Double Click on Method IF_HTTP_EXTENSION~HANDLER and add the following code.
METHOD if_http_extension~handle_request.* Variables
TYPES : BEGIN OF local_type_response,
success TYPE string,
msg TYPE string,
data TYPE ztt_testuser,
END OF local_type_response.* Objects
DATA : lo_contact TYPE REF TO zapi_test,
lo_json_serializer TYPE REF TO cl_trex_json_serializer. ” Standard Functon of SAP.* Internal Tables
DATA : lt_contacts TYPE STANDARD TABLE OF zapi_testuser.* Structures
DATA : ls_contact TYPE zapi_testuser,
ls_response TYPE local_type_response.* Method Variables
DATA : l_rc TYPE i,
l_json TYPE string.DATA : l_verb TYPE string,
l_path_info TYPE string,
l_resource TYPE string,
l_param_1 TYPE string,
l_param_2 TYPE string.* Retriving the request methods ( POST, GET, PUT and DELETE)
l_verb = server->request->get_header_field( name = ‘~request_method’).* Retriving the parameters passed in the URL
l_path_info = server->request->get_header_field( name = ‘~path_info’).SHIFT l_path_info LEFT BY 1 PLACES.
SPLIT l_path_info AT ‘/’ INTO l_resource
l_param_1
l_param_2.* Only Methods GET, POST, PUT and DELETE are allowed
IF ( l_verb NE ‘GET’ ) AND ( l_verb NE ‘POST’ ) AND ( l_verb NE ‘PUT’ ) AND ( l_verb NE ‘DELETE’).
* For any other method the service should return the error code 405
CALL METHOD server->response->set_status( code = ‘405’
reason = ‘Method not allowed’).CALL METHOD server->response->set_header_field( name = ‘Allow’
value = ‘POST, GET, PUT, DELETE’).EXIT.
ENDIF.* Method Action to execute.
CASE l_verb.
WHEN ‘POST’. “(Create)
*Call create method to the model.
CLEAR : ls_contact,
ls_response,
l_rc.* Retrive form DATA
ls_contact-email = server->request->get_form_field(’email’).
ls_contact-firstname = server->request->get_form_field(‘firstname’).
ls_contact-lastname = server->request->get_form_field(‘lastname’).CREATE OBJECT lo_contact.
* Calling the Method for create
CALL METHOD lo_contact->CREATE
EXPORTING
i_s_contact = ls_contact
IMPORTING
e_rc = l_rc.
IF l_rc IS INITIAL.
ls_response-success = ‘true’.
ls_response-msg = ‘User Created Successfully.’.
ELSE.
ls_response-success = ‘false’.
ls_response-msg = lo_contact->get_message( ).
ENDIF.* Returing the form data received back to the client.
APPEND ls_contact TO ls_response-data.WHEN ‘GET’.
* Method tto Read DataCLEAR : ls_contact,
ls_response.CREATE OBJECT lo_contact.
* Retrive contacts email passed in the url to read.
ls_contact-email = l_param_1.* Retrive query string data
ls_contact-firstname = server->request->get_form_field(‘firstname’).
ls_contact-lastname = server->request->get_form_field(‘lastname’).* Read Data
CALL METHOD lo_contact->read
EXPORTING
i_s_contact = ls_contact
IMPORTING
e_t_contacts = lt_contacts.IF NOT lt_contacts[] IS INITIAL.
ls_response-success = ‘true’.
ls_response-data[] = lt_contacts[].
ELSE.
ls_response-success = ‘false’.
ls_response-msg = lo_contact->get_message( ).ENDIF.
WHEN ‘PUT’.
* Method to Update the Data,CLEAR : ls_contact,
ls_response,
l_rc.* Retrive the email address passed from the URL.
ls_contact-email = l_param_1.* Retrive query string data
ls_contact-firstname = server->request->get_form_field(‘firstname’).
ls_contact-lastname = server->request->get_form_field(‘lastname’).
CREATE OBJECT lo_contact.* Method to call update data.
CALL METHOD lo_contact->update
EXPORTING
i_s_contact = ls_contact
IMPORTING
e_rc = l_rc.IF l_rc IS INITIAL.
ls_response-success = ‘true’.
ls_response-msg = ‘User Data Update Successfully.’.
ELSE.
ls_response-success = ‘false’.
ls_response-msg = lo_contact->get_message( ).
ENDIF.* Returing the form data received back to the client.
APPEND ls_contact TO ls_response-data.WHEN ‘DELETE’.
* Method to delete user data.
CLEAR : ls_contact,
ls_response,
l_rc.* Retrive email address pass from URL to delete.
ls_contact-email = l_param_1.CREATE OBJECT lo_contact.
CALL METHOD lo_contact->delete
EXPORTING
i_s_contact = ls_contact
IMPORTING
e_rc = l_rc.IF l_rc IS INITIAL.
ls_response-success = ‘true’.
ls_response-msg = ‘User data deleted successfully.’.
ELSE.
ls_response-success = ‘false’.
ls_response-msg = lo_contact->get_message( ).
ENDIF.*Returing data to the client.
APPEND ls_contact TO ls_response-data.
ENDCASE.* DATA Serializing to JSON
CREATE OBJECT lo_json_serializer
EXPORTING
DATA = ls_response.CALL METHOD lo_json_serializer->serialize.
CALL METHOD lo_json_serializer->get_data
RECEIVING
rval = l_json.*Sets the content type of the response
CALL METHOD server->response->set_header_field( name = ‘Content-Type’
value = ‘application/json; charset=iso-8859-1’ ).*Retruen the results to JSON format
CALL METHOD server->response->set_cdata( data = l_json ).
ENDMETHOD.
Step 6: Add Methods and their respcetive parameters. Here we add CREATE, READ, UPDATE, DELETE and GET_MESSAGE Methods.

Step 7 : Add the following codes Code to the Methods to Update, Delete and Add Data. These are basic codes to read, update, insert and delete data. Activate Object now. We have completed the class object for POST, GET, PUT and DELETE Methods for API.
Code to Create / Insert Data
method CREATE.
DATA : l_email TYPE ZAPI_TESTUSER-email.
* Check the data exists.
select SINGLE email from ZAPI_TESTUSER INTO l_email
where email = i_s_contact-email.if sy-subrc = 0.
e_rc = 4.
me->status = ‘Contact already exists’.
exit.
endif.* Create data
INSERT ZAPI_TESTUSER from i_s_contact.
e_rc = sy-subrc.endmethod.
Code to Read Data
METHOD read.
DATA : l_cond(72) TYPE c,
lt_cond LIKE STANDARD TABLE OF l_cond.CLEAR : l_cond, lt_cond[].
IF NOT i_s_contact-email IS INITIAL.
CONCATENATE ‘EMAIL = ”’ i_s_contact-email ”” INTO l_cond.
APPEND l_cond TO lt_cond.
ENDIF.IF NOT i_s_contact-firstname IS INITIAL.
IF l_cond IS INITIAL.
CONCATENATE ‘FIRSTNAME LIKE ”%’ i_s_contact-firstname ‘%”’ INTO l_cond.
ELSE.
CONCATENATE ‘OR FIRSTNAME LIKE ”%’ i_s_contact-firstname ‘%”’ INTO l_cond.
ENDIF.
ENDIF.IF NOT i_s_contact-lastname IS INITIAL.
IF l_cond IS INITIAL.
CONCATENATE ‘LASTNAME LIKE ”%’ i_s_contact-lastname ‘%”’ INTO l_cond.
ELSE.
CONCATENATE ‘OR LASTNAME LIKE ”%’ i_s_contact-lastname ‘%”’ INTO l_cond.
ENDIF.
ENDIF.IF NOT lt_cond[] IS INITIAL.
SELECT email firstname lastname FROM zapi_testuser
INTO CORRESPONDING FIELDS OF TABLE e_t_contacts
WHERE (lt_cond).ELSE.
SELECT email firstname lastname FROM zapi_testuser
INTO CORRESPONDING FIELDS OF TABLE e_t_contacts.ENDIF.
ENDMETHOD.
Code to Update Data
method UPDATE.
* You can add validations here if required.UPDATE ZAPI_TESTUSER from i_s_contact.
e_rc = sy-subrc.endmethod.
Code to Delete Data from SAP
method DELETE.
DELETE FROM ZAPI_TESTUSER where email = i_s_contact-email.
endmethod.
Code to get Status Message
method GET_MESSAGE.
r_msg = me->status.endmethod.
In this section we learned to create SAP Table, Table Type and methods to READ, INSERT, DELETE data from and to SAP through JSON.
You can watch the complete video tutorial also.
In our Next Section Create a service in SAP using T-Code SICF, we learn to create a SAP service to display JSON data in web browser.
Thank you for the good tutorial.
My SAP demo system does not have json serializable class cl_trex_json_serialize as it it is old version i.e. version 700. Could you please suggest some way out for similar serialization
Please post your question in http://www.seevablog.com/community/sap-forum/
Could you please post similar tutorial for IDOC
Please wait for few days. We will post IDOC tutorial.